ChemInventory's API enables you to build scripts to interact with the data saved in your inventory, such as to execute actions according to a programmable schedule or to integrate the system with external software.
API Authentication
ChemInventory's API is URL-based, where requests are sent to various HTTPS endpoints to retrieve or modify data in your inventory. The body of each request includes an authtoken
parameter to authenticate access to the system.
These authentication tokens are tied to a given user account, with any actions carried out by that key authenticated and executed as if it were that user who initiated them. Crucially, this means that the functionality and actions available to each token are governed by the level of access the user has — that is, their privilege level . You can therefore configure token permissions through the associated user account.
It is possible to create multiple tokens for your inventory, each tied to a different user account or tied to the same account but used for different purposes. When creating a token, you can give the token a name to describe its purpose (e.g. "Weekly Export/Backup"). This can assist with token management in future, such as when revoking or rotating tokens.
Generating authentication tokens
Authentication tokens can be generated by users with Group Administrator privileges from the Inventory Management page. Open the Inventory Tools menu and click on API Tokens item.
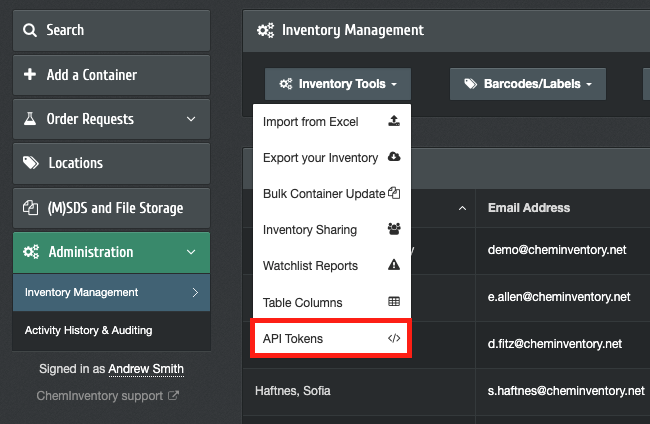
When creating a new token, you will be prompted to enter a name for the token and select the user account the token is tied to. After the token has been generated, you will be able to view the token only once. You must handle this token securely as it gives full authenticated access to your inventory.
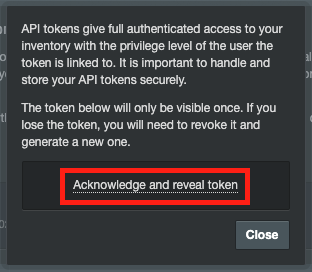
It is not possible to view raw authentication tokens again, outside this initial generation process. If you lose a token, then you will need to revoke it and generate a new one.
Accessing the API
Querying an endpoint
ChemInventory's API can be accessed from the programming language of your choice, by sending a POST request to an HTTPS endpoint. Data included with your query, such as your authtoken
, should be formatted as application/json
.
Below is an example search query, written in NodeJS using the Axios HTTP client library:
const axios = require('axios'); axios({ method: "post", url: "https://app.cheminventory.net/api/search/execute", data: { "authtoken": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx", "inventory": 9, "type": "name", "contents": "dipea" } }) .then(response => console.log(response))
And the equivalent in Python, using the requests library:
import requests myRequest = requests.post( "https://app.cheminventory.net/api/search/execute", json = { "authtoken": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx", "inventory": 9, "type": "name", "contents": "dipea" } ) print(myRequest.text)
Your authtoken
must be included in every query that you send to an endpoint. If you do not include a valid token, your request will be rejected.
Structure of response
All queries to endpoints return a standardized response, consisting of a JSON-formatted string with two properties: status
and data
. The status
property indicates whether the request was processed successfully (the server will return "success" or "error") while the data
property will include data retrieved from the server, or will be an empty array, depending on what endpoint was queried.
A response for the example search query above could be:
{ "status": "success", "data": { "orders": [], "containers": [ { "id": 856461, "name": "N,N-diisopropyl ethylamine 99%", "location": 40032, "size": "50", "unitid": 1, "unit": "mL", "substance": 4454, "cas": "7087-68-5", "isomericsmiles": "CCN(C(C)C)C(C)C", "needsretrieval": 0, "comments": "Red label on bottle.", "barcode": "AEF01028", "supplier": "Aldrich", "dateacquired": "2016-06-30", "inventory": 9, "inventoryname": "Demo Group", "sitename": "Demo Site", "locationtext": "Cupboards > Cupboard 1" } ] } }
In the event of an "error" status
response, the data
property will provide more information about the issue that has arisen.
URL Endpoints
Each of the endpoints listed below are relative to the root URL of your ChemInventory installation, plus an /api
suffix. For most users, the API can be accessed at https://app.cheminventory.net/api
. However, this URL may differ for you if you are covered by a Dedicated Cloud or self-hosted ChemInventory installation.
The 'Body Parameters' section under each endpoint describes the information which needs to be sent in your request body alongside the authtoken
parameter. The 'Returns' section describes how information is returned from the endpoint.
/barcodes/assigned
Retrieves information listing all assigned barcodes. Only available to users with Group Administrator privileges.
/barcodes/duplicates/check
Gets details of existing container(s)/location(s)/user(s) which have already been assigned the barcode supplied in request. Takes into account site/enterprise level settings, if applicable.
/barcodes/duplicates/getall
Retrieves information for duplicate barcodes in the user's inventory. Takes into account site/enterprise level settings, if applicable. Available to users with administrator privileges only.
/barcodes/generator/createvalues
Returns an array of potential new barcode values. Will update barcode prefix saved for inventory, if permitted, using supplied prefix parameter.
/container/add
Adds new container(s) to an inventory. The locationid
and substanceid
values supplied for each object in the data
array must be the same (endpoint will take values in data[0]
and use for all subsequent entries). In effect this means that one endpoint call can be used to add multiple containers of the same substance to the same location.
An example container addition process can be found in the Example Workflows section at the bottom of this page.
/container/delete
Deletes specified container(s).
/container/getsubstance
Retrieves substance information using details supplied. If not already present in database, adds new substance record. If substanceid
supplied, retrieves substance info for specified substance.
/container/ghs/delete
Deletes a GHS entry.
/container/ghs/save
Either updates an existing entry or adds a new ghs entry (if entryid
is 0).
/container/information/gethistory
Retrieves full history & audit logs for a container.
/container/information/load
Retrieves all information saved for specified container, including custom fields.
/container/information/save
Updates value saved for a field of a container.
/container/information/togglerecycling
Toggles recycling status of container, if recycling is enabled for the inventory's site. Only available within site and enterprise licenses.
/container/move
Moves specified container(s) to specified location.
/container/recentlyadded
Returns details of containers added to the user's inventory within last 30 days. Only available to users with Group Administrator privileges.
/customfields/delete
Deletes specified custom field.
/customfields/get
Retrieves full list of custom fields available to user.
/customfields/save
Either updates an existing custom field or creates a new custom field (if id
is 0).
/filestore/addsubstancelink
Adds a new substance link to a file.
/filestore/createcategory
Creates a new file category.
/filestore/deletecategory
Deletes specified file category.
/filestore/deletefile
Deletes a file.
/filestore/download
Retrieves a URL where the file can be downloaded from. The URL is time-sensitive, and will expire after 30 minutes.
/filestore/getlinkedfiles
Retrieves files that are linked to specified substance.
/filestore/getsubstancelinks
Gets list of substances that file is currently linked to.
/filestore/load
Retrieves file storage information available to user, including file categories and files themselves. For users within a site/enterprise structure, organization-wide file store data are also retrieved.
/filestore/removesubstancelink
Removes a substance link from a file.
/filestore/renamecategory
Renames specified file category.
/filestore/renamefile
Renames a file.
/filestore/updatefilecategory
Updates category for specified file.
/general/exportbyid
Retrieves all information saved for specified containers.
/general/getdetails
Gets general inventory and user information about the account associated with the API key. This includes IDs of the user account and inventory, along with suppliers, units, custom fields and locations. Effectively a 'one stop shop' for all data needed for subsequent requests.
/general/retrievesubstanceinfo
Sources chemical information for a new substance, including chemical structure and synonyms. Only applies to substances with a CAS number (i.e. commercially-available).
/general/units
Gets information of container size units that already exist in user's inventory.
/general/updatestructure
Updates structure for specified substance. If the structure matches an already-existing substance, the existing ID will be returned.
/inventorymanagement/audithistory/load
Retrieves activity logs for user's inventory within specified date range.
/inventorymanagement/deletedcontainers/export
Exports full information about all deleted containers, including custom fields.
/inventorymanagement/deletedcontainers/get
Retrieves a summary list of all deleted container records in user's inventory.
/inventorymanagement/deletedcontainers/restore
Restores a deleted container, including its parent location(s).
/inventorymanagement/export
Exports full information about all containers in user's inventory, including custom fields.
/inventorymanagement/users/add
Creates a new user account in specified inventory.
/inventorymanagement/users/disable
Removes access for specified user from specified inventory.
/inventorymanagement/users/list
Retrieves user accounts within specified. Available to users with administrator privileges only.
/inventorymanagement/users/resetpassword
Resets the password for specified user. Cannot be used with SSO accounts.
/inventorymanagement/users/update
Updates details of user account (email, name, etc.).
/location/containers/delete
Deletes all containers in specified location.
/location/containers/get
Retrieves containers in specified location.
/location/containers/move
Moves all containers from origin location to destination location.
/location/load
Retrieves all locations in user's inventory, including IDs.
/mycollection/add
Adds specified container(s) to user's 'My Collection'.
/mycollection/addbybarcode
Adds containers to user's 'My Collection', identifying container(s) by barcode(s).
/mycollection/deleteall
Delete all containers in user's 'My Collection'.
/mycollection/load
Retrieves containers currently saved in user's 'My Collection'.
/mycollection/moveall
Moves all containers in user's 'My Collection' to a specified location.
/mycollection/remove
Removes specified container from user's 'My Collection'.
/mycollection/removeall
Removes all containers from user's 'My Collection'.
/navbar/switchinventory
Switches the active inventory for user, if user has access to more than one inventory. Note: this endpoint should rarely be used. It is only available to users within site/enterprise licenses.
/order/addcontainer
Adds container to inventory, marks order request as arrived. Note: most details for corresponding container record are retrieved automatically from order request (size, supplier, etc.).
/order/delete
Deletes specified order request.
/order/edit
Updates specified order request.
/order/load
Retrieves current active order requests in user's inventory.
/order/request
Creates a new order request in user's inventory.
/order/toggleordered
Toggles specified request's ordered status.
/search/execute
Executes search against specified inventory. Processes any results found using the 'limit search to' as saved against user account (this may strip results). The user's 'limit search to' can only be modified through ChemInventory's user interface.
/search/loadadvancedsearch
Loads advanced search options, following format to meet requirements of https://querybuilder.js.org/.
/watchlist/checksubstance
Checks whether a specified substance appears on any watchlists.
/watchlist/delete
Deletes a custom watchlist.
/watchlist/generatereport
Generates watchlist report for specified watchlist.
/watchlist/load
Retrieves watchlists accessible by user (including custom watchlists defined at the site and enterprise levels, if applicable).
/watchlist/save
Updates the details of a watchlist, or creates a new one if id
is 0.
Example Workflows
The sections below show some example query/request workflows for common activities in ChemInventory. We will add sections over time based on feedback and queries our support team receives.